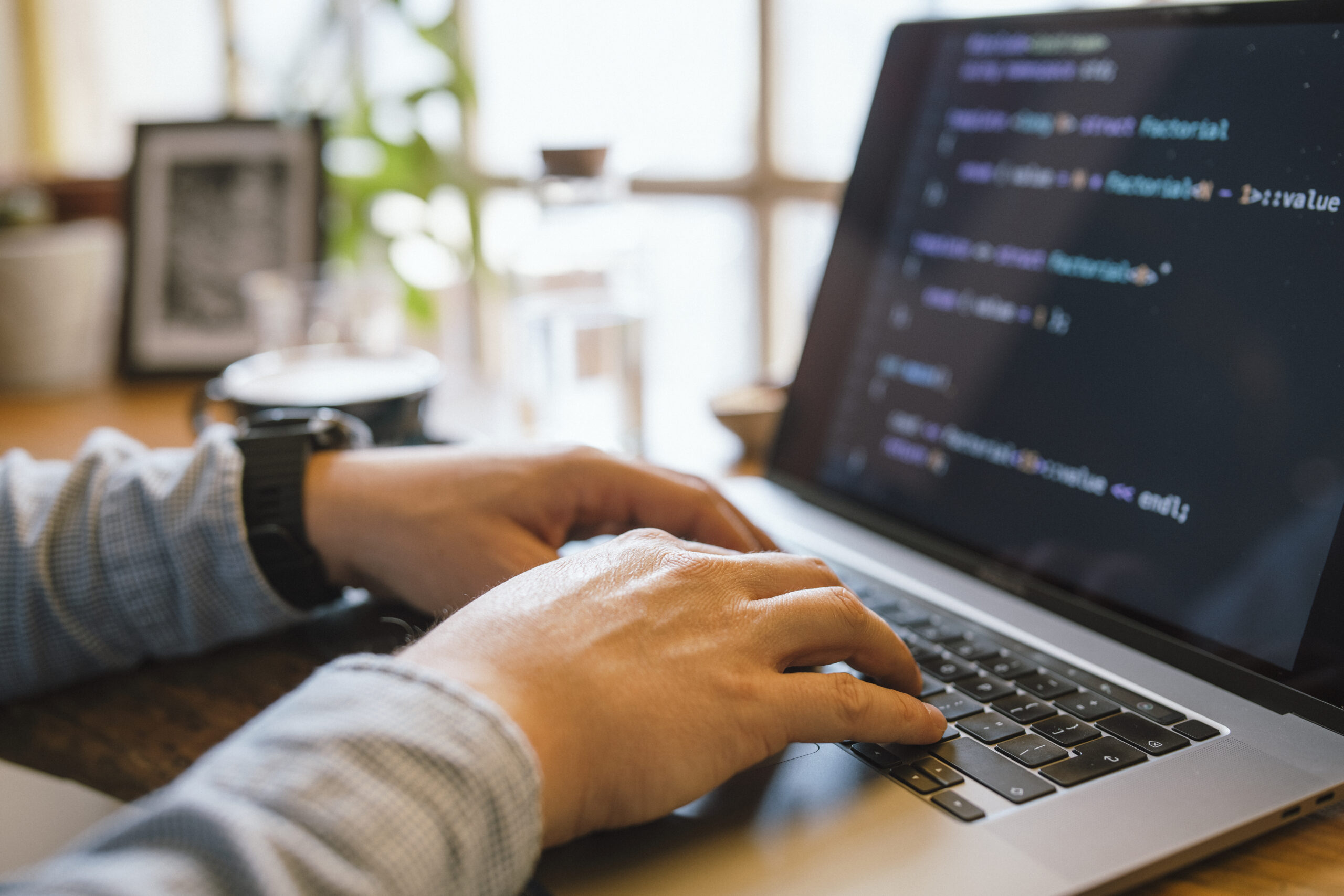
Debugging is Probably the most crucial — but normally disregarded — competencies in a developer’s toolkit. It is not almost correcting damaged code; it’s about comprehending how and why factors go Completely wrong, and Understanding to Consider methodically to unravel issues proficiently. Whether you're a novice or maybe a seasoned developer, sharpening your debugging capabilities can help save several hours of irritation and dramatically enhance your productivity. Listed here are various tactics that can help builders level up their debugging recreation by me, Gustavo Woltmann.
Grasp Your Equipment
On the list of fastest ways builders can elevate their debugging skills is by mastering the tools they use everyday. Though crafting code is one A part of development, knowing how to connect with it properly for the duration of execution is equally crucial. Contemporary improvement environments come Geared up with highly effective debugging capabilities — but numerous builders only scratch the floor of what these instruments can do.
Acquire, by way of example, an Integrated Development Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources allow you to established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code on the fly. When applied appropriately, they Permit you to observe precisely how your code behaves all through execution, that's invaluable for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-close developers. They assist you to inspect the DOM, keep an eye on community requests, check out real-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can change disheartening UI concerns into workable duties.
For backend or procedure-degree developers, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Command in excess of running processes and memory administration. Studying these instruments can have a steeper Understanding curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become cozy with Model Command methods like Git to comprehend code heritage, find the exact second bugs have been launched, and isolate problematic variations.
Ultimately, mastering your resources usually means going past default options and shortcuts — it’s about producing an personal expertise in your improvement surroundings to ensure when difficulties crop up, you’re not lost at midnight. The higher you recognize your instruments, the greater time you could spend solving the particular trouble rather than fumbling through the process.
Reproduce the issue
Just about the most vital — and often disregarded — methods in successful debugging is reproducing the trouble. Just before jumping into the code or making guesses, builders will need to make a steady atmosphere or scenario where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of possibility, usually leading to squandered time and fragile code alterations.
The first step in reproducing a problem is accumulating as much context as possible. Check with inquiries like: What actions triggered The problem? Which environment was it in — enhancement, staging, or generation? Are there any logs, screenshots, or error messages? The greater depth you've, the a lot easier it will become to isolate the exact disorders beneath which the bug occurs.
When you finally’ve collected more than enough data, attempt to recreate the problem in your neighborhood atmosphere. This may imply inputting a similar facts, simulating related person interactions, or mimicking technique states. If the issue appears intermittently, think about crafting automated checks that replicate the edge cases or point out transitions involved. These assessments not just assistance expose the trouble but also avert regressions Down the road.
Often, The difficulty could be ecosystem-particular — it would materialize only on particular running systems, browsers, or beneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating such bugs.
Reproducing the issue isn’t merely a action — it’s a mindset. It calls for endurance, observation, and a methodical strategy. But when you finally can regularly recreate the bug, you happen to be already halfway to repairing it. That has a reproducible scenario, You can utilize your debugging instruments more efficiently, examination probable fixes properly, and communicate much more Obviously using your staff or buyers. It turns an summary criticism right into a concrete obstacle — Which’s where by builders prosper.
Go through and Realize the Mistake Messages
Error messages are sometimes the most useful clues a developer has when a thing goes Completely wrong. Rather then looking at them as discouraging interruptions, builders need to learn to treat mistake messages as immediate communications from your method. They often tell you what precisely took place, the place it occurred, and occasionally even why it happened — if you know the way to interpret them.
Start off by reading through the information thoroughly and in full. Lots of builders, particularly when below time pressure, look at the initial line and instantly get started generating assumptions. But deeper from the error stack or logs may lie the genuine root result in. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Crack the mistake down into parts. Could it be a syntax error, a runtime exception, or possibly a logic error? Will it point to a certain file and line number? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging course of action.
Some errors are obscure or generic, As well as in These situations, it’s crucial to examine the context where the mistake occurred. Examine linked log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings both. These generally precede larger sized problems and provide hints about likely bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a extra efficient and assured developer.
Use Logging Properly
Logging is The most highly effective applications in a developer’s debugging toolkit. When utilized properly, it provides true-time insights into how an software behaves, supporting you fully grasp what’s occurring underneath the hood without needing to pause execution or step with the code line by line.
A great logging technique starts with understanding what to log and at what level. Common logging levels include DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for thorough diagnostic data for the duration of growth, Data for basic activities (like productive commence-ups), WARN for potential issues that don’t crack the appliance, ERROR for precise challenges, and Deadly when the system can’t go on.
Prevent flooding your logs with extreme or irrelevant information. An excessive amount of logging can obscure important messages and decelerate your program. Concentrate on key situations, state improvements, input/output values, and critical final decision points in your code.
Structure your log messages clearly and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace problems in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily worthwhile in production environments the place stepping by code isn’t doable.
Furthermore, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. Having a very well-believed-out logging tactic, you are able to decrease the time it's going to take to spot difficulties, achieve further visibility into your programs, and improve the Total maintainability and trustworthiness of one's code.
Assume Like a Detective
Debugging is not only a complex task—it's a sort of investigation. To effectively recognize and deal with bugs, builders should technique the procedure similar to a detective solving a thriller. This mentality helps break down elaborate problems into manageable areas and observe clues logically to uncover the root trigger.
Start off by collecting proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency troubles. The same as a detective surveys against the law scene, obtain just as much suitable information and facts as you can without jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent picture of what’s happening.
Next, form hypotheses. Ask your self: What might be creating this behavior? Have any changes recently been built into the codebase? Has this challenge transpired just before below comparable circumstances? The intention will be to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble inside a managed setting. Should you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Enable the outcome lead you nearer to the truth.
Fork out close notice to modest details. Bugs generally conceal within the the very least expected sites—like a lacking semicolon, an off-by-a single mistake, or even a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely understanding it. Momentary fixes may cover the actual difficulty, just for it to resurface later.
And finally, continue to keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future troubles and help Other folks understand your reasoning.
By pondering similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be more practical at uncovering hidden concerns in advanced systems.
Publish Checks
Writing exams is among the simplest tips on how to enhance your debugging capabilities and Over-all development effectiveness. Exams not simply assistance capture bugs early but additionally serve as a safety Web that gives you confidence when creating adjustments to the codebase. A very well-analyzed software is much easier to debug mainly because it allows you to pinpoint precisely in which and when an issue occurs.
Start with unit tests, which focus on individual functions or modules. These small, isolated checks can quickly expose whether a selected bit of logic is Performing as predicted. Each time a examination fails, you right away know exactly where to appear, considerably decreasing the time used debugging. Device exams are Specifically helpful for catching regression bugs—issues that reappear after Beforehand currently being mounted.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These check here assistance be sure that different elements of your application get the job done jointly easily. They’re especially useful for catching bugs that come about in intricate methods with multiple parts or providers interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to think critically about your code. To check a attribute properly, you require to know its inputs, predicted outputs, and edge instances. This volume of knowing naturally qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the exam fails regularly, you may focus on repairing the bug and enjoy your test pass when The problem is fixed. This approach makes sure that a similar bug doesn’t return in the future.
In a nutshell, crafting tests turns debugging from a discouraging guessing game into a structured and predictable approach—serving to you capture much more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the problem—staring at your display for several hours, seeking solution right after Resolution. But Among the most underrated debugging applications is simply stepping absent. Having breaks helps you reset your mind, reduce aggravation, and often see the issue from a new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain turns into significantly less effective at issue-solving. A brief stroll, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of an issue after they've taken the perfect time to disconnect, permitting their subconscious operate inside the track record.
Breaks also help reduce burnout, Specially for the duration of more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent means that you can return with renewed Vitality and a clearer mentality. You could possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Specifically less than tight deadlines, but it really really causes more quickly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a sensible strategy. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be an opportunity to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, every one can instruct you some thing worthwhile for those who make an effort to reflect and examine what went Mistaken.
Start out by inquiring yourself a couple of crucial issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught previously with greater procedures like device tests, code reviews, or logging? The answers usually reveal blind spots with your workflow or knowledge and help you Develop stronger coding routines moving forward.
Documenting bugs will also be a great behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and Anything you acquired. Eventually, you’ll begin to see designs—recurring concerns or frequent blunders—that you could proactively steer clear of.
In team environments, sharing Anything you've figured out from the bug along with your peers is usually Primarily highly effective. No matter whether it’s through a Slack information, a brief compose-up, or a quick know-how-sharing session, supporting Other individuals avoid the similar concern boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your frame of mind from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial parts of your progress journey. In any case, a few of the most effective developers are not those who write great code, but people who continuously study from their errors.
Eventually, Each and every bug you take care of adds a different layer for your ability established. So up coming time you squash a bug, have a moment to mirror—you’ll occur away a smarter, additional capable developer as a consequence of it.
Conclusion
Bettering your debugging skills will take time, exercise, and patience — even so the payoff is huge. It helps make you a far more efficient, assured, and able developer. Another time you're knee-deep in the mysterious bug, remember: debugging isn’t a chore — it’s a chance to be better at Everything you do.